Writing a “Hello World” Web App with NodeJS and Express
Mar 19, 2018
In this post, I will show you how to write a basic web app with nodejs and Express.
Initial Steps
First, download and install nodejs in your system, then create a new directory where the application files will reside. Next, switch to this new directory:
$ mkdir hello-world-app
$ cd hello-world-app
We now use the Node Package Manager (npm) to install the Express framework.
Note: npm comes bundled with NodeJS (select the LTS version of the NodeJS download).
First, use the npm init command
to create a package.json
file. This file will contain a list of all dependencies required by the project (if you are familiar with Maven in Java, this corresponds to the pom.xml
file). In our case, the only dependency is the Express package:
$ npm init
When running this command, you can press RETURN to accept the default values when prompted. Make note of the entry point:
option, the default value is index.js
, this is the name of the main application file.
Now, install Express using the npm install
command, and use the --save
option to automatically save it to the dependencies list (inside the package.json
file):
$ npm install express --save
Creating the Nodejs App
We are now ready to start writing our simple nodejs web app. Next, create another directory named views under our current working directory. Our app will serve a static HTML file from this directory when it receives an HTTP GET request to the root route (/).
$ mkdir views
$ touch views/index.html
The index.html
file is a simple HTML document with an <h1>
tag:
<!DOCTYPE html>
<html>
<head>
<title>My Express App</title>
</head>
<body>
<h1>Hello World!</h1>
</body>
</html>
Then create the index.js
file inside your working directory. Open the file in your favorite editor and type the following code:
1 var express = require('express'),
2 app = express(),
3 port = process.env.PORT || 3000;
4
5 app.use(express.static(__dirname + '/views'));
6
7 app.get("/", function(req, res){
8 res.send('index.html');
9 });
10
11 app.listen(port, function(){
12 console.log('Server running on port ' + port);
13 });
In lines 1 and 2 above, we load the Express module and create an instance named app
. Line 3 declares a port
variable, this will be the port on which the app will listen for requests. If the environment variable PORT
is empty, then the default port 3000 is used instead. The use()
method in line 5 allows us to serve static content located in the /views
directory.
The get()
method in line 7 handles HTTP GET
requests. The first argument (“/”) specifies the request's endpoint, and the second one is the handler function that serves the request. The req
and res
arguments to the handler function are objects that wrap nodejs’ HTTP request and response objects. They are used to extract request information and set response data respectively (we can name these arguments anything we want, but req and res are used conventionally). We use the send()
method (line 8) of the res
object, this method sends the HTTP response. In our app, we are sending the index.html
file as a response to the GET request. The response object contains many useful methods. I recommend taking a look at the Express API documentation.
Finally, in line 11, we use the listen()
method to tell our app to start listening to requests on the specified port.
Running the App
To run the app, on your working directory enter command node index.js
. This will start the application and begin listening for requests.
$ node index.js
A message like this will be returned:
Server running on port 3000
Now open a web browser and go to http://localhost:3000. We should see the simple HTML file with the "Hello World" title:
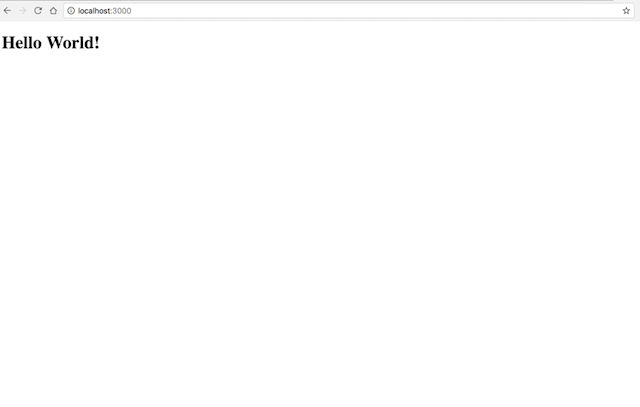
Conclusion
We have written a very basic web application with Express and nodejs. However, they provide many more features to build more complex applications. Hopefully, this post helped to introduce some of the basics of Express and nodejs.